자바에서 사용자가 숫자를 입력하면 팩토리얼 값을 구해주는 함수를 만들어보자
그 전에 팩토리얼에 대해 알아보자
팩토리얼이란 ‘수를 단계적으로 곱한다.’는 뜻으로 숫자 옆에 !표를 붙어서 표현한다.
팩토리얼을 구하는 것은 아래와 같이 표현할 수 있다.
3!=3*2*1 =6
4!=4*3*2*1 = 24
5!=5*4*3*2*1= 120
1. for문을 이용해서 만들기
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("팩토리얼 값을 원하는 숫자를 입력 하세요");
System.out.print(">>> ");
int n = Integer.parseInt(sc.nextLine());
long result = 1l;
for (int i = 1; i <= n; i++) {
result *= i;
}
System.out.println( n +"! = " + result);
}
|
cs |
2. 재귀함수를 이용해서 만들기
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("팩토리얼 값을 원하는 숫자를 입력 하세요");
System.out.print(">>> ");
int n = Integer.parseInt(sc.nextLine());
System.out.println(n +"! = " + factorial(n));
}
static int factorial(int n) {
if (n == 1) {
return 1;
}
return n * factorial(n - 1);
}
|
cs |
두 가지 방법 모두 아래 사진처럼 결과가 나온다
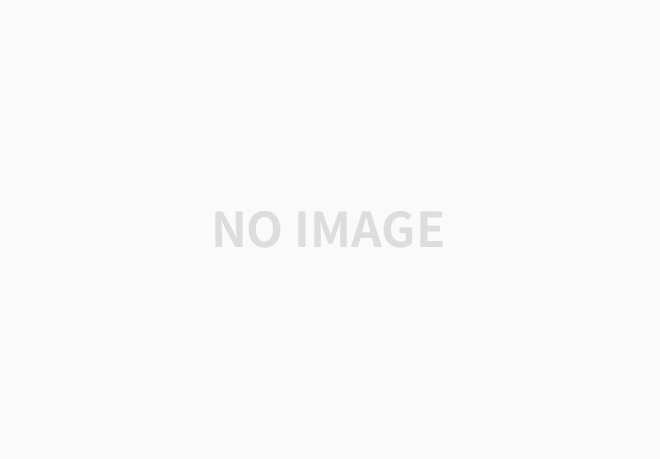
만약 사용자에게 입력받지 않으려면 아래와 같이 아예 값을 설정해주면 된다. 현재는 n에 5라는 숫자를 넣어주었다.
1
2
3
4
5
6
7
8
9
10
11
|
public static void main(String[] args) {
int n = 5;
System.out.println(n +"! = " + factorial(n));
}
static int factorial(int n) {
if (n == 1) {
return 1;
}
return n * factorial(n - 1);
}
|
cs |
출력 결과는 아래와 같다
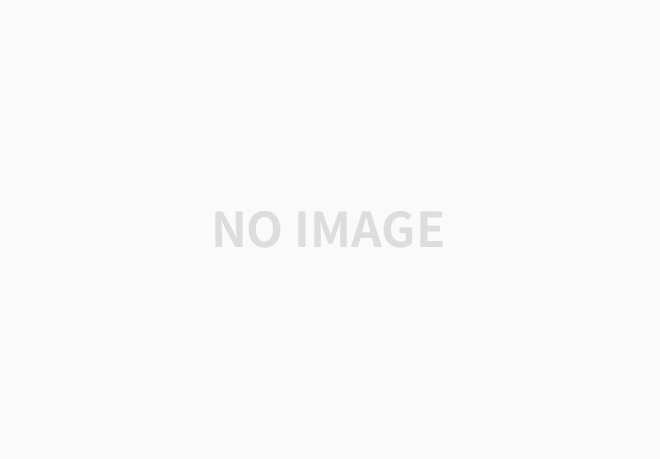
'JAVA' 카테고리의 다른 글
JAVA - 숫자를 입력 받아 구구단 출력하기 (0) | 2022.05.31 |
---|---|
JAVA - 피보나치 수열의 값 구하기 (0) | 2022.05.31 |
JAVA - 동전의 사용 개수를 구하는 문제 (500,100,50,10원) (0) | 2022.05.24 |
JAVA - ArrayList를 알고리즘(버블정렬)과 메소드로 정렬해보자 (0) | 2022.05.23 |
JAVA - 콘솔창에 달력 출력하기 (0) | 2022.05.19 |
댓글